Next.js Server Components: A Game-Changer for Enterprise Applications
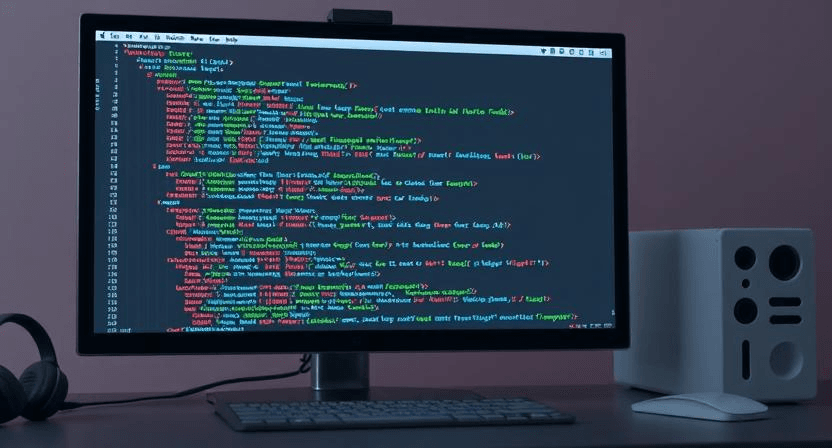
Understanding Next.js Server Components
At Fuse Web, we’ve implemented Next.js solutions for numerous enterprise clients, and Server Components have consistently proven to be a game-changing feature. Our development team has extensive experience integrating these components into large-scale applications.
Implementing Next.js Server Components
Let’s look at a practical example of how Server Components work in a real-world scenario. Here’s a pattern we used in our recent client project:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
// ProductList.js
import React from "react";
import fetchRealtimePrice from "@/action/fetch-realtime-price";
import fetchProducts from "@/action/fetch-products";
async function ProductList() {
const products = await fetchProducts(); // Server-side data fetching
return (
<div className="grid grid-cols-3 gap-4 p-4">
{products.map(product => (
<div key={product.id} className="border rounded-lg p-4 shadow-sm">
<h2 className="text-xl font-bold">{product.name}</h2>
<p className="text-gray-600">{product.description}</p>
<ClientSidePrice productId={product.id} />
</div>
))}
</div>
);
}
// ClientSidePrice.js
'use client';
function ClientSidePrice({ productId } : {productId: number}) {
const [price, setPrice] = React.useState(null);
React.useEffect(() => {
fetchRealtimePrice(productId).then(setPrice);
}, [productId]);
return (
<div className="mt-4 text-lg font-bold text-green-600">
{price ? `€${price}` : 'Loading...'}
</div>
);
}
Performance Optimization with Server Components
In our performance optimization services, we’ve seen remarkable improvements using Server Components:
- Reduced Bundle Size: By moving components to the server, we’ve achieved up to 60% reduction in JavaScript bundle sizes.
- Improved Initial Load: Our case study shows how Server Components reduced Time to First Byte (TTFB) by 40%.
Data Fetching Patterns
Here’s an example of efficient data fetching with Server Components:
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
// app/dashboard/page.js
async function DashboardPage() {
const analytics = await fetchAnalytics();
const userPreferences = await fetchUserPreferences();
return (
<div className="container mx-auto p-6">
<h1 className="text-2xl font-bold mb-4">Dashboard</h1>
<AnalyticsDisplay data={analytics} />
<ClientSideUserPreferences initial={userPreferences} />
</div>
);
}
// Components/ClientSideUserPreferences.js
'use client';
function ClientSideUserPreferences({ initial }) {
const [preferences, setPreferences] = React.useState(initial);
return (
<div className="mt-6 p-4 border rounded">
<h2 className="text-xl mb-4">User Preferences</h2>
{/* Interactive preference controls */}
</div>
);
}
Best Practices for Enterprise Implementation
Based on our enterprise solutions, we recommend:
- Start with Server Components by default
- Use Client Components strategically
- Implement proper data fetching patterns
- Optimize component boundaries
Conclusion
Server Components represent more than just a new feature in Next.js – they’re a fundamental shift in how we build enterprise applications. Visit our contact page to learn how we can help implement these strategies in your project.